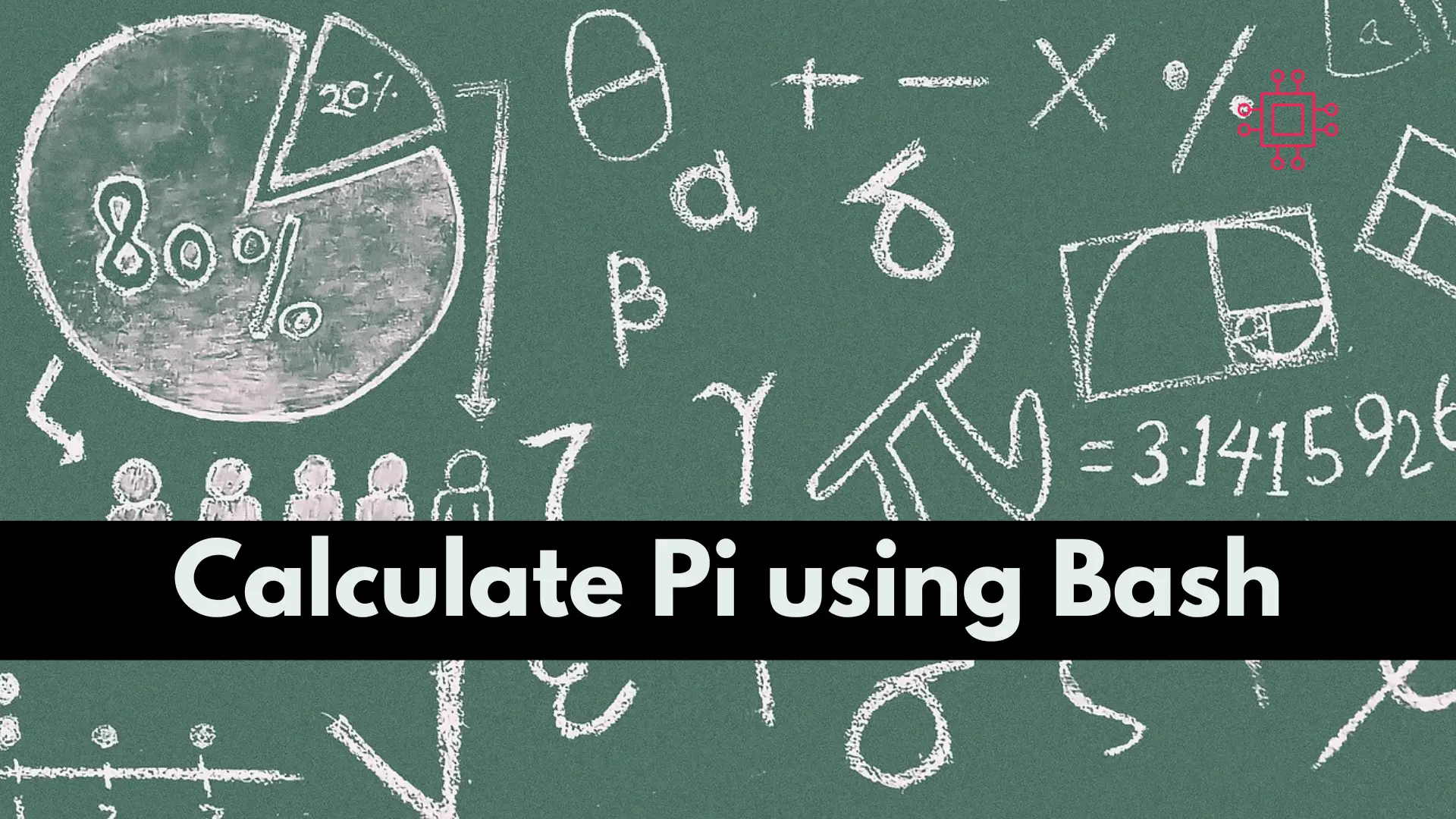
To calculate pi using a Bash script, you can use the bc command, which is a tool that can perform arithmetic operations in the terminal.
Are you preparing for the RHCSA 9 exam and want to master the essential skill of shell scripting? Discover 10 practical shell scripts that can automate tasks in Linux and help you excel in the exam. From conditional code execution to processing script inputs and outputs, these scripts cover all the key areas and will give you a competitive edge in the RHCSA 9 exam.
Aspiring Red Hat Certified System Administrator (RHCSA) candidates need to have a good command of shell scripting, which is an essential part of the RHCSA 9 exam. Shell scripting is a valuable tool for automating tasks in Linux, allowing users to write programs that can perform repetitive tasks, handle file input/output, and process data. In this article, we will explore 10 simple shell scripts that are perfect for practicing RHCSA 9 exam skills. We will break down the scripts into the following sections: conditionally execute code, use of looping constructs, process script inputs, and process the output of shell commands.
Conditionally executing code is a critical aspect of shell scripting. The if statement is the most basic conditional statement, which allows you to test if a condition is true or false and perform different actions based on the result.
The first script checks if a file exists and returns a message accordingly. If the file does not exist, the script will prompt the user to create it.
#!/bin/bash
FILE="/path/to/your/file.txt"
if [ -f "$FILE" ]; then
echo "$FILE exists."
else
echo "$FILE does not exist."
read -p "Do you want to create it? (y/n) " ANSWER
if [[ $ANSWER == "y" || $ANSWER == "Y" ]]; then
touch $FILE
echo "File created successfully."
else
echo "File creation canceled."
fi
fi
The second script prompts the user to enter a number between 1 and 10 and checks if the input is valid. If the input is not a number or is outside the range, the script will display an error message.
#!/bin/bash
read -p "Enter a number between 1 and 10: " NUM
if [[ "$NUM" =~ ^[0-9]+$ ]]; then
if [ $NUM -ge 1 -a $NUM -le 10 ]; then
echo "Valid input."
else
echo "Input out of range."
fi
else
echo "Invalid input."
fi
Looping constructs are used to repeat a set of commands multiple times. The most commonly used looping constructs in shell scripting are for loops and while loops.
The third script uses a for loop to process multiple files in a directory. In this example, the script looks for all the files with the .txt extension in the /path/to/your/directory directory and performs a simple text search on each file.
#!/bin/bash
for FILE in /path/to/your/directory/*.txt
do
echo "Processing file: $FILE"
grep "search term" $FILE
done
The fourth script uses a while loop to process command line input. The script reads command line arguments and prints them to the console until there are no more arguments left.
#!/bin/bash
ARGS=("$@")
COUNT=0
while [ "$COUNT" -lt "$#" ]; do
echo "Argument $COUNT: ${ARGS[$COUNT]}"
COUNT=$((COUNT+1))
done
Shell scripts can accept input from the user, which can be processed by the script. Script inputs are stored in variables called positional parameters, which are represented by $1, $2, $3, and so on.
The fifth script processes user input using positional parameters. The script prompts the user to enter their name and age and then displays the information in a formatted message.
#!/bin/bash
echo "Enter your name: "
read NAME
echo "Enter your age: "
read AGE
echo "Hello $NAME, you are $AGE years old."
The sixth script uses the getopts command to process user input with options. The script accepts three options: -h (display help), -f (set file name), and -d (set directory). The script displays the help message if the -h option is used or sets the file name and directory if the -f and -d options are used.
#!/bin/bash
while getopts ":hf:d:" opt; do
case $opt in
h)
echo "Usage: script.sh [-h] [-f filename] [-d directory]"
exit 0
;;
f)
FILENAME=$OPTARG
;;
d)
DIRECTORY=$OPTARG
;;
\?)
echo "Invalid option: -$OPTARG" >&2
exit 1
;;
esac
done
echo "File name: $FILENAME"
echo "Directory: $DIRECTORY"
Shell scripts can execute shell commands and process the output of those commands. This allows scripts to automate tasks that would normally require manual intervention.
The seventh script uses the grep command to process the output of the ps command. The script displays a list of all running processes that contain the word “apache“.
#!/bin/bash
ps aux | grep apache
The eighth script uses the awk command to process the output of the df command. The script displays the total size, used space, and available space for the /dev/sda1 partition.
#!/bin/bash
df -h /dev/sda1 | awk '{print "Total: " $2 "\nUsed: " $3 "\nAvailable: " $4}'
The ninth script uses the find command to count the number of files in a directory (excluding sub-directories).
#!/bin/bash
DIRECTORY="/path/to/your/directory"
COUNT=$(find "$DIRECTORY" -maxdepth 1 -type f | wc -l)
echo "Number of files in directory: $COUNT"
The tenth script creates a backup of a file by appending the current date and time to the file name and saving it in a backup directory.
#!/bin/bash
FILE="/path/to/your/file.txt"
BACKUP_DIR="/path/to/your/backup/directory"
BACKUP_FILE="${BACKUP_DIR}/$(date +"%Y%m%d_%H%M%S")_$(basename "$FILE")"
cp "$FILE" "$BACKUP_FILE"
echo "Backup created at: $BACKUP_FILE"
Shell scripting is a critical skill for RHCSA candidates, and these 10 simple shell scripts will help you practice the key aspects of shell scripting needed for the exam. These scripts cover conditionally executing code, using looping constructs, processing script inputs, and processing the output of shell commands. With practice and familiarity with these scripts, you will be well-prepared for the RHCSA 9 exam.
While these scripts may seem straightforward, mastering shell scripting takes time and practice. It’s important to keep practicing and experimenting with different scripts to gain more experience and improve your skills. With dedication and persistence, you’ll be on your way to becoming an expert in shell scripting and achieving your RHCSA certification.
Was the article helpful to you? If so, leave us a comment below. We value your feedback!
Related Posts
To calculate pi using a Bash script, you can use the bc command, which is a tool that can perform arithmetic operations in the terminal.
In this article, we will review installing and using Vi or Vim, a versatile and powerful text editor commonly found in Unix-based operating systems like
In this create simple shell scripts section of the RHCSA9 Exam Series, we’ll review some topics and labs that may show up on the exam.