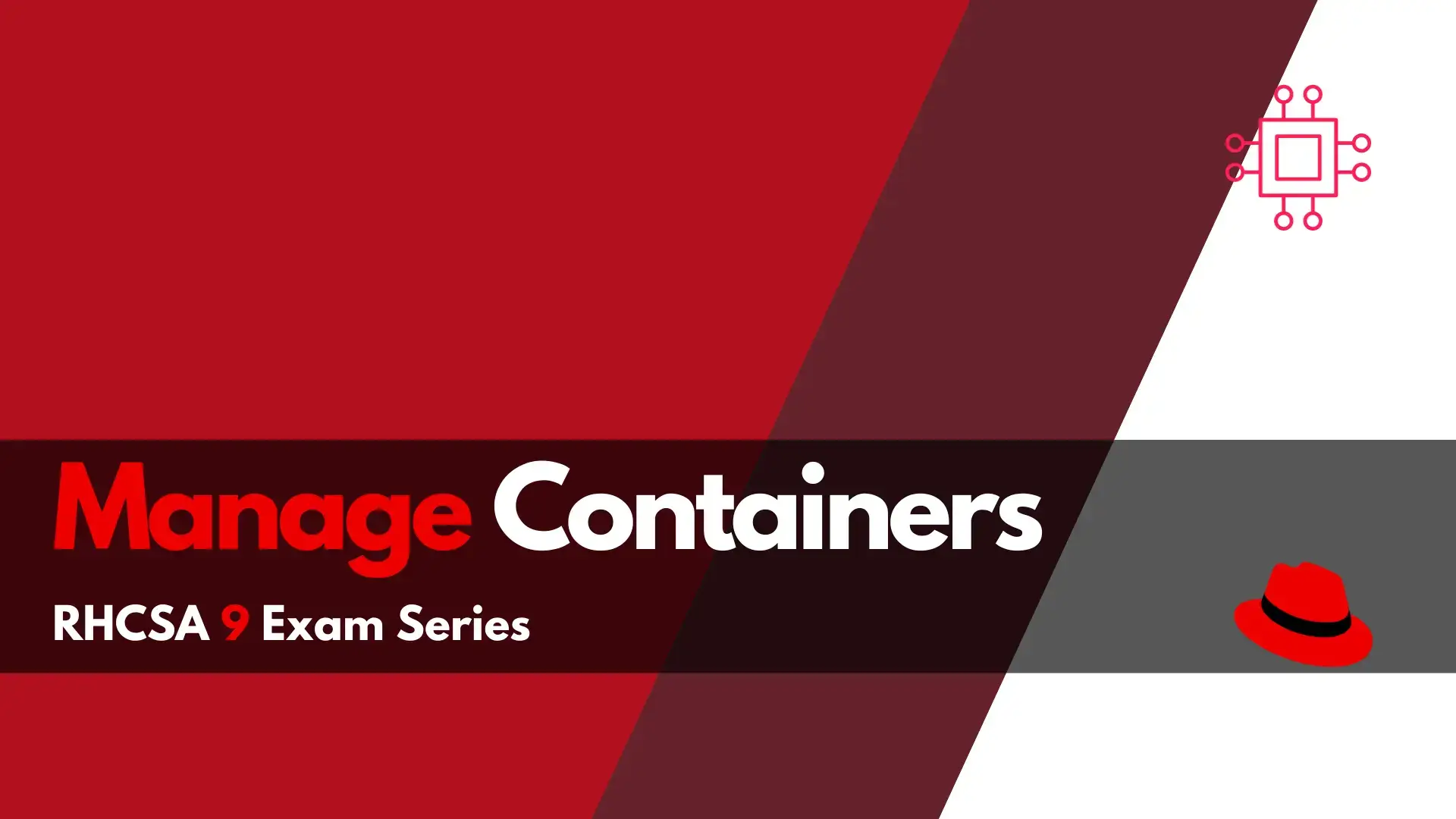
Looking to enhance your Linux skillset? RHCSA 9 Container Management Skills introduces a new section on container management, making it a must-have competency for any
In this create simple shell scripts section of the RHCSA9 Exam Series, we’ll review some topics and labs that may show up on the exam.
Our aim in writing this article is to provide you with a comprehensive understanding of the knowledge, tasks, and skills required to pass the RHCSA 9 exam. Your success on the exam is dependent on your own efforts and dedication. To achieve confidence in completing all the RHCSA exam objectives, we strongly encourage you to practice the labs multiple times until you feel confident in your abilities. With diligent effort and practice, you’ll be well-prepared for the RHCSA exam and equipped with valuable skills for your career as a Linux professional.
Here are a few basic bash script examples that may appear on the RHCSA 9 exam. Don’t worry too much about this section, as you won’t be expected to write extensive scripts to complete complex tasks. The RHCSA exam simply assesses your basic or fundamental understanding of shell scripting concepts. As a result, scripting tasks will typically involve incorporating simple Linux command-line (CLI) commands into an executable *.sh or *.bash file.
This script takes a file name as an argument and displays its contents to the screen.
#!/bin/bash
if [ -e $1 ]; then
cat $1
else
echo "File not found"
fi
This script takes a file name as an argument and counts the number of lines in the file.
#!/bin/bash
if [ -e $1 ]; then
lines=$(wc -l $1 | awk '{print $1}')
echo "$1 has $lines lines"
else
echo "File not found"
fi
This script takes a file name as an argument and creates a backup of the file with a timestamp appended to its name.
#!/bin/bash
if [ -e $1 ]; then
timestamp=$(date +%Y%m%d%H%M%S)
cp $1 $1.$timestamp
echo "Backup of $1 created at $1.$timestamp"
else
echo "File not found"
fi
This script takes a directory name as an argument and checks the disk usage of the directory.
if [ -d $1 ]; then
du -sh $1
else
echo "Directory not found"
fi
This script takes a username as an argument and creates a new user account with that name.
#!/bin/bash
if [ $# -ne 1 ]; then
echo "Usage: $0 "
exit 1
fi
if id $1 >/dev/null 2>&1; then
echo "User $1 already exists"
else
useradd $1
echo "User $1 created"
fi
This section includes both questions and answers related to bash scripting tasks. You’ll be given tasks to complete, and the solutions will be provided for your reference. However, we recommend that you attempt to complete the tasks on your own before consulting the answer or solutions section. It’s important to put in enough effort to solve the task independently before checking the solution to get the most out of this section.
Create a script that takes a username as an argument and deletes the user account with that name.
#!/bin/bash
if [ $# -ne 1 ];then
echo "Usage: $0 <username>"
exit 1
fi
if id $1 >/dev/null 2>&1;then
userdel -r $1
echo "User $1 deleted"
else
echo "User $1 not found"
fi
Create a script that takes a service name as an argument and checks if the service is currently running.
#!/bin/bash
if [ $# -ne 1 ]; then
echo "Usage: $0 <service_name>"
exit 1
fi
if systemctl is-active --quiet $1; then
echo "$1 is running"
else
echo "$1 is not running"
fi
Create a script that takes a service name as an argument and restarts the service.
#!/bin/bash
if [ $# -ne 1 ]; then
echo "Usage: $0 <service_name>"
exit 1fi
systemctl restart $1
echo "$1 restarted"
Write a bash script that takes a file name as an argument and compresses the file using gzip.
#!/bin/bash
if [ $# -ne 1 ]; then
echo "Usage: $0 <file_name>"
exit 1
fi
if [ -e $1 ]; then
gzip $1
echo "$1 compressed"else
echo "File not found"
fi
Write a bash script that checks the disk space usage of the system and reports the top 5 directories using the most disk space.
#!/bin/bash
echo "Top 5 directories by disk space usage:"
du -hs /* 2>/dev/null | sort -rh | head -n 5
Congratulations on completing the Create Simple Shell Scripts section of the RHCSA9 exam series!
The simple Bash scripts presented in this section are just a few examples of what you might encounter on the RHCSA 9 exam. Keep in mind that there are multiple ways to write a bash script, but the end result must match the expected outcome. By practicing these scripts and gaining a better understanding of how they work, you can increase your confidence in Bash scripting and be better prepared for the exam.
If you found the tasks challenging, don’t worry! Keep practicing until you can complete them more quickly.
Did you find this article helpful? If so, please leave us some feedback in the comments section. We value your input!
Related Posts
Looking to enhance your Linux skillset? RHCSA 9 Container Management Skills introduces a new section on container management, making it a must-have competency for any
Are you ready to take on the RHCSA 9 exam? Our comprehensive guide on creating and configuring file systems in RHCSA will equip you with
Want to pass the RHCSA9 exam? This section of the RHCSA9 Exam Series: Operate Running Systems will review how to operate running systems–a key skill