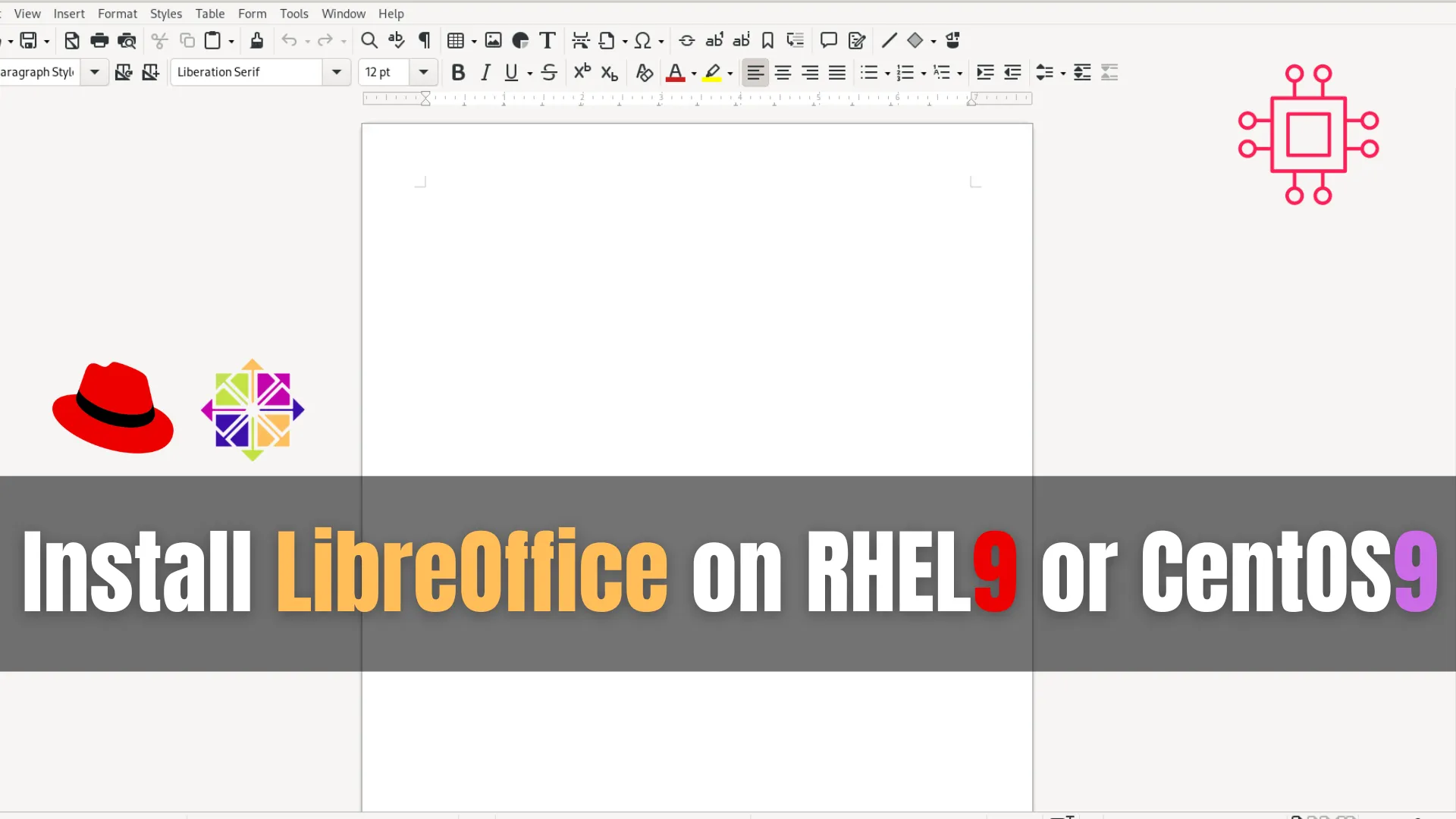
In this guide, we’ll walk you through the step-by-step process of installing LibreOffice on RHEL 9 or CentOS 9. Table of Contents Introduction Are you
Learn how to combine multiple PDFs into one using Python. This comprehensive guide covers step-by-step instructions, CLI examples, and troubleshooting tips for merging PDFs with Python’s PyPDF2 library.
In the digital world, managing files efficiently is key to improving productivity and organizing your data. One common task that many users face is merging multiple PDF files into a single document. Whether you are a student compiling research papers, a business professional combining reports, or a developer automating document management, knowing how to combine PDFs using Python can save time and effort.
In this blog post, we will walk you through a detailed step-by-step guide on how to combine multiple PDF files into a single PDF document using Python (specifically Python 3). This guide is aimed at both beginners and developers who want to learn how to merge PDFs programmatically. We will also cover the prerequisites, the best libraries for PDF manipulation, and provide practical examples.
Why Merge PDFs? |
Merging PDFs has several practical benefits:
|
|
|
Before we dive into the code, let’s ensure you have the necessary tools installed on your system. To merge PDFs with Python, we will use the PyPDF2 library, which is a popular tool for working with PDF files.
Install PyPDF2 |
First, you need to install the PyPDF2 library if you haven’t already. Open your terminal or command prompt and run the following command:
pip3 install PyPDF2
This will install PyPDF2, a Python library that allows you to manipulate PDF files, including merging multiple PDFs into a single document.
💡The |
Photo by admingeek from Infotechys
Now that the prerequisites are covered, let’s look at the Python script you need to merge multiple PDFs. This script combines all PDF files in a specified directory into a single PDF file. Using your preferred text-editor, open a file called combine_pdf.py:
sudo vim combine_pdf.py
Copy and paste the following into the file:
import os
import sys
from PyPDF2 import PdfMerger
def combine_pdfs(directory, output_file):
# Create a PdfMerger object
merger = PdfMerger()
# Get all the files in the directory
for filename in os.listdir(directory):
# Check if the file is a PDF
if filename.endswith('.pdf'):
pdf_path = os.path.join(directory, filename)
merger.append(pdf_path)
# Write the combined PDF to the output file
with open(output_file, 'wb') as output_pdf:
merger.write(output_pdf)
print(f"Combined PDF saved as {output_file}")
if __name__ == "__main__":
# Ensure that the directory path is passed as an argument
if len(sys.argv) != 2:
print("Usage: python combine_pdfs.py ")
sys.exit(1)
# Get the PDF directory path from the command-line argument
pdf_directory = sys.argv[1]
# Check if the provided directory exists
if not os.path.isdir(pdf_directory):
print(f"Error: The directory '{pdf_directory}' does not exist.")
sys.exit(1)
# Define the output PDF file name
output_pdf = 'combined_output.pdf' # You can customize this
combine_pdfs(pdf_directory, output_pdf)
Save and exit the file. The next section gives a brief overview of this code actually does.
How the Code Works |
Let’s break down the code:
Imports: We use the os module for interacting with the operating system (e.g., listing files in a directory) and the PyPDF2 module to handle PDF merging. |
combine_pdfs() function: This function takes two arguments: |
directory
: The path to the folder containing the PDFs you want to merge.output_file
: The path and filename for the final merged PDF.It creates a PdfMerger object to handle the merging process and appends each PDF file from the directory to the merger. Finally, the merged file is written to the specified output location.
Command-Line Argument: The script accepts the path to the directory containing PDFs as a command-line argument, allowing you to run the script for different directories without modifying the code. |
Directory Validation: The script checks if the provided directory exists and contains PDF files. If not, it displays an error message. |
Running the scripts |
To run the script, you will need to use the terminal (command prompt on Windows, terminal on macOS/Linux). Here’s how to execute the script:
python3 combine_pdfs.py /path/to/your/pdf/directory
Replace /path/to/your/pdf/directory
with the actual directory path where your PDF files are stored. After running the script, the PDFs in that directory will be merged into a single PDF file named combined_output.pdf.
Example Command |
python3 combine_pdfs.py /Users/john/Documents/pdfs
This command will take all PDF files in the /Users/john/Documents/pdfs directory and merge them into combined_output.pdf
.
There are several libraries available in Python for working with PDF files. PyPDF2 is one of the most popular and versatile libraries for merging, splitting, and manipulating PDFs. Here’s a quick overview of some common PDF libraries in Python:
Library | Description | Use Cases |
---|---|---|
PyPDF2 | A library for PDF manipulation that supports merging, splitting, and rotating PDFs. | Merging, splitting, rotating PDFs |
reportlab | A library for creating PDFs from scratch, useful for generating PDFs. | PDF generation, custom content creation |
pdfminer | A library for extracting text and information from PDF files. | Text extraction, data mining |
PyMuPDF | A powerful library for reading and writing PDF files. | Advanced PDF manipulation |
Here are some common issues users may encounter when merging PDFs using the Python script:
1. Permission Error: |
This error may occur if you do not have the necessary permissions to read or write files in the specified directory. Ensure that your user account has read and write permissions for the directory and PDF files.
2. Missing PyPDF2 Library |
If you run the script and see an error saying that PyPDF2 is not found, you may need to install it first by running:
pip install PyPDF2
pip3 install PyPDF2 # Python Version 3+
3. Incorrect Directory Path |
If the directory you provide does not exist, the script will terminate with an error. Double-check the path to ensure it’s correct, and make sure the directory contains PDF files.
Merging PDFs into a single document can significantly streamline your workflow, whether you’re handling documents for work, study, or personal projects. Using Python’s PyPDF2 library to automate the process allows you to save time and ensure consistency. With the steps provided in this guide, you can easily merge multiple PDFs into one file using just a few lines of code.
By following this tutorial, you’ll not only be able to merge PDFs but also gain valuable experience working with Python’s PDF manipulation libraries, preparing you for more advanced document handling tasks.
Did you find this article helpful? Your feedback is greatly appreciated! Feel free to share this post with others who might find it useful and leave your thoughts in the comments below.
In this guide, we’ll walk you through the step-by-step process of installing LibreOffice on RHEL 9 or CentOS 9. Table of Contents Introduction Are you
In this article, we will learn how to install Python SciPy on CentOS9 or RHEL9, the powerful library for advanced mathematics, statistics, and optimization! Table
Scientific computing using Python refers to the use of the Python programming language and its associated libraries to solve scientific problems. Scientific computing is the