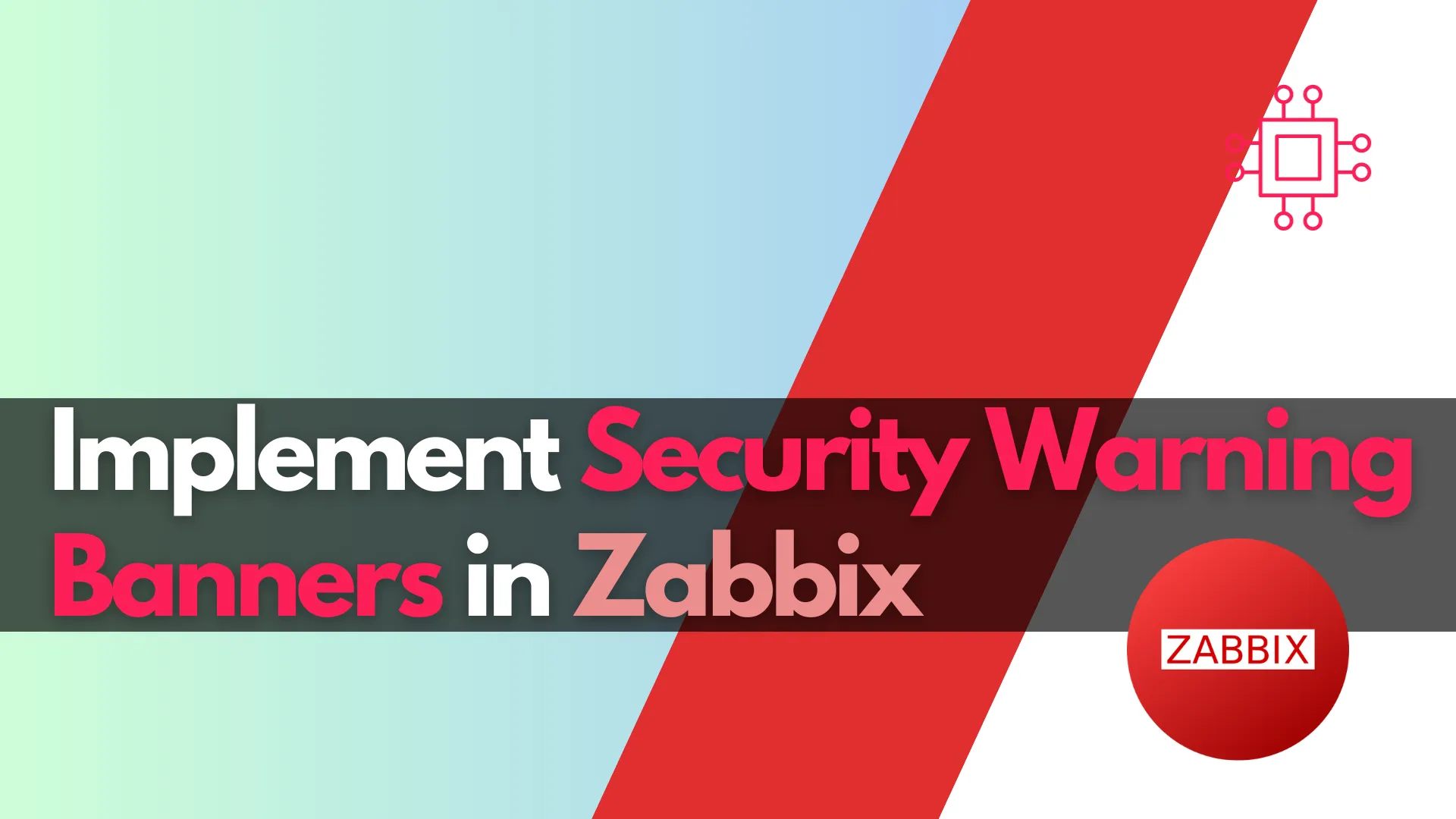
In this article, we will explore how to implement security warning banners in Zabbix to enhance compliance and user awareness. This step-by-step guide covers creating
Learn how to automate adding hosts to Zabbix using Bash script. This comprehensive guide covers script functions, examples, and best practices to streamline your IT monitoring process. Optimize your workflow and reduce errors with our step-by-step instructions!
In the realm of IT monitoring, Zabbix stands out as a powerful open-source solution. However, efficiently adding hosts can be a time-consuming process if done manually. In this blog post, we’ll explore how to automate the addition of hosts into Zabbix using a Bash script, designed for ease of use and flexibility.
Why Automate Host Addition? |
Before diving into the script, ensure you have:
Required Database Credentials |
Update the Database entries in the script to match your environment.
# Zabbix PostgreSQL database credentials
DB_USER="zabbix"
DB_PASSWORD="zabbix"
DB_NAME="zabbix"
DB_HOST="192.168.1.4"
DB_PORT="5432"
Make sure the host running the script has permission to access the database. You can do this by updating the pg_hba.conf
file to include the IP address of the host.
host zabbix zabbix /32 trust
Here’s the full Bash script inventory_update.sh
:
#!/bin/bash
# inventory_update.sh
# Purpose: This script adds hosts and respective IP addresses to the Zabbix database. It takes
# the path to a file with a list of hostnames and IP addresses as arguments.
# Zabbix PostgreSQL database credentials
DB_USER="zabbix"
DB_PASSWORD="zabbix"
DB_NAME="zabbix"
DB_HOST="192.168.1.4"
DB_PORT="5432"
# Function to check if a file exists
check_file_exists() {
if [ ! -f "$1" ]; then
echo "File $1 does not exist."
exit 1
fi
}
# Function to check if a host already exists in the database
host_exists() {
local hostname="$1"
local existing_host
existing_host=$(PGPASSWORD="$DB_PASSWORD" psql -U "$DB_USER" -h "$DB_HOST" -p "$DB_PORT" -d "$DB_NAME" -t -c \
"SELECT hostid FROM hosts WHERE host = '$hostname';" | sed 's/^ *//;s/ *$//')
if [ -n "$existing_host" ]; then
echo -e "Host \033[1;31m$hostname\033[0m already exists with host ID: \033[1;31m$existing_host\033[0m."; sleep 0.5
return 0
else
return 1
fi
}
# Function to get the next host ID
get_next_host_id() {
local next_host_id
next_host_id=$(PGPASSWORD="$DB_PASSWORD" psql -U "$DB_USER" -h "$DB_HOST" -p "$DB_PORT" -d "$DB_NAME" -t -c \
"SELECT COALESCE(MAX(hostid), 0) + 1 FROM hosts;" | sed 's/^ *//;s/ *$//')
if [ -z "$next_host_id" ]; then
echo "Failed to retrieve the next host ID."
exit 1
fi
echo "$next_host_id"
}
# Function to get the next interface ID
get_next_interface_id() {
local next_int_id
next_int_id=$(PGPASSWORD="$DB_PASSWORD" psql -U "$DB_USER" -h "$DB_HOST" -p "$DB_PORT" -d "$DB_NAME" -t -c \
"SELECT COALESCE(MAX(interfaceid), 0) + 1 FROM interface;" | sed 's/^ *//;s/ *$//')
if [ -z "$next_int_id" ]; then
echo "Failed to retrieve the next interface ID."
exit 1
fi
echo "$next_int_id"
}
# Function to add host to Zabbix
add_host() {
local hostname="$1"
local ip_address="$2"
local host_id
local interface_id
echo "Adding host $hostname with IP $ip_address..."
# Check if the host already exists
if host_exists "$hostname"; then
echo "Skipping addition of $hostname as it already exists."
return 0
fi
# Get the next host ID
host_id=$(get_next_host_id)
if [ -z "$host_id" ]; then
echo "Failed to get the next host ID."
return 1
fi
echo "Next host ID: $host_id"
local sql_host="INSERT INTO hosts (hostid, host, name, status) VALUES ('$host_id', '$hostname', '$hostname', 0) RETURNING hostid;"
local result
result=$(PGPASSWORD="$DB_PASSWORD" psql -U "$DB_USER" -h "$DB_HOST" -p "$DB_PORT" -d "$DB_NAME" -t -c "$sql_host" 2>&1 | sed 's/^ *//;s/ *$//')
if [[ "$result" == *"ERROR"* || -z "$result" ]]; then
echo "Failed to add host $hostname. SQL Result: $result"
return 1
fi
# Get the next interface ID
interface_id=$(get_next_interface_id)
if [ -z "$interface_id" ]; then
echo "Failed to get the next interface ID."
return 1
fi
echo "Next interface ID: $interface_id"
# Insert the IP address into the interface table
local sql_interface="INSERT INTO interface (interfaceid, hostid, main, ip, dns, port) VALUES ('$interface_id', '$host_id','1', '$ip_address', '$hostname', 10050);"
result=$(PGPASSWORD="$DB_PASSWORD" psql -U "$DB_USER" -h "$DB_HOST" -p "$DB_PORT" -d "$DB_NAME" -c "$sql_interface" 2>&1)
if [[ "$result" == *"ERROR"* ]]; then
echo "Failed to add IP address $ip_address for host ID $host_id. SQL Result: $result"
return 1
fi
echo "Successfully added IP address $ip_address for host $hostname (host ID: $host_id, interface ID: $interface_id)."
return 0
}
# Main function to process the hosts file
process_hosts_file() {
local hosts_file="$1"
while IFS= read -r line; do
# Skip empty lines or lines starting with #
[[ -z "$line" || "$line" =~ ^# ]] && continue
# Extract the hostname and IP address
hostname=$(echo "$line" | awk '{print $1}')
ip_address=$(echo "$line" | awk '{print $2}')
# Check if both hostname and IP are provided
if [ -z "$hostname" ] || [ -z "$ip_address" ]; then
echo "Invalid entry: $line. Skipping..."
continue
fi
# Add host and its IP address
add_host "$hostname" "$ip_address"
if [ $? -eq 0 ]; then
echo -e "Successfully processed host \033[1;32m$hostname\033[0m with IP \033[1;32m$ip_address\033[0m."; sleep 0.5
echo -e "";
fi
done < "$hosts_file"
}
# Main script execution
main() {
# Check if the user provided the correct number of arguments
if [ $# -ne 1 ]; then
echo "Usage: $0 "
exit 1
fi
# File containing hosts and IPs
local hosts_file="$1"
# Ensure the file exists
check_file_exists "$hosts_file"
# Process the hosts file
process_hosts_file "$hosts_file"
}
# Call the main function with all script arguments
main "$@"
The script consists of six(6) key functions:
Function | Description |
---|---|
check_file_exists | Verifies if the provided hosts file exists. |
host_exists | Checks if a host already exists in the database. |
get_next_host_id | Retrieves the next available host ID. |
get_next_interface_id | Retrieves the next available interface ID. |
add_host | Main function to add a host and its IP address to Zabbix. |
process_hosts_file | Processes the input file containing host details. |
Check if File Exists |
check_file_exists() {
if [ ! -f "$1" ]; then
echo "File $1 does not exist."
exit 1
fi
}
This function checks if the specified hosts file exists and exits if it doesn’t.
Check if Host Already Exists |
host_exists() {
local hostname="$1"
local existing_host
existing_host=$(PGPASSWORD="$DB_PASSWORD" psql -U "$DB_USER" -h "$DB_HOST" -p "$DB_PORT" -d "$DB_NAME" -t -c \
"SELECT hostid FROM hosts WHERE host = '$hostname';" | sed 's/^ *//;s/ *$//')
if [ -n "$existing_host" ]; then
echo -e "Host \033[1;31m$hostname\033[0m already exists."
return 0
else
return 1
fi
}
This function queries the database to check if the host is already present, preventing duplicates.
Get Next Host ID |
get_next_host_id() {
local next_host_id
next_host_id=$(PGPASSWORD="$DB_PASSWORD" psql -U "$DB_USER" -h "$DB_HOST" -p "$DB_PORT" -d "$DB_NAME" -t -c \
"SELECT COALESCE(MAX(hostid), 0) + 1 FROM hosts;" | sed 's/^ *//;s/ *$//')
echo "$next_host_id"
}
This function retrieves the next available host ID by finding the maximum current ID and incrementing it.
Add Host to Zabbix |
add_host() {
local hostname="$1"
local ip_address="$2"
local host_id
local interface_id
# Check if the host already exists
if host_exists "$hostname"; then
echo "Skipping addition of $hostname as it already exists."
return 0
fi
host_id=$(get_next_host_id)
# Insert host into database...
}
The add_host
function coordinates the addition of a new host and its interface, ensuring all necessary checks are performed before inserting into the database.
The main
function checks for the correct number of arguments and processes the provided hosts file.
# Main script execution
main() {
# Check if the user provided the correct number of arguments
if [ $# -ne 1 ]; then
echo "Usage: $0 "
exit 1
fi
# File containing hosts and IPs
local hosts_file="$1"
# Ensure the file exists
check_file_exists "$hosts_file"
# Process the hosts file
process_hosts_file "$hosts_file"
}
# Call the main function with all script arguments
main "$@"
Example Input File |
The hosts file should be formatted like this (assuming we have a file called hosts_file.txt
and populated it with the following):
redhat-srv01.dev.naijalabs.net 192.168.1.181
redhat-srv02.dev.naijalabs.net 192.168.1.204
redhat-srv03.dev.naijalabs.net 192.168.1.224
redhat-srv04.dev.naijalabs.net 192.168.1.46
redhat-srv05.dev.naijalabs.net 192.168.1.182
centos-labs06.dev.naijalabs.net 192.168.1.205
centos-labs07.dev.naijalabs.net 192.168.1.225
redhat-labs08.dev.naijalabs.net 192.168.1.47
redhat-labs09.dev.naijalabs.net 192.168.1.183
redhat-labs10.dev.naijalabs.net 192.168.1.206
redhat-labs11.dev.naijalabs.net 192.168.1.226
ubuntu-node12.dev.naijalabs.net 192.168.1.48
ubuntu-node13.dev.naijalabs.net 192.168.1.184
redhat-labs14.dev.naijalabs.net 192.168.1.207
redhat-labs15.dev.naijalabs.net 192.168.1.227
redhat-labs16.dev.naijalabs.net 192.168.1.49
redhat-labs17.dev.naijalabs.net 192.168.1.185
redhat-labs18.dev.naijalabs.net 192.168.1.208
redhat-labs19.dev.naijalabs.net 192.168.1.228
redhat-labs20.dev.naijalabs.net 192.168.1.50
redhat-labs21.dev.naijalabs.net 192.168.1.186
redhat-labs22.dev.naijalabs.net 192.168.1.209
redhat-labs23.dev.naijalabs.net 192.168.1.229
redhat-labs24.dev.naijalabs.net 192.168.1.51
redhat-labs25.dev.naijalabs.net 192.168.1.187
To execute the script, use the following command:
$ ./inventory_update.sh /path/to/hosts_file.txt
Adding host redhat-srv01.dev.naijalabs.net with IP 192.168.1.181...
Next host ID: 10639
Next interface ID: 37
Successfully added IP address 192.168.1.181 for host redhat-srv01.dev.naijalabs.net (host ID: 10639, interface ID: 37).
Successfully processed host redhat-srv01.dev.naijalabs.net with IP 192.168.1.181.
Adding host redhat-srv02.dev.naijalabs.net with IP 192.168.1.204...
Next host ID: 10640
Next interface ID: 38
Successfully added IP address 192.168.1.204 for host redhat-srv02.dev.naijalabs.net (host ID: 10640, interface ID: 38).
Successfully processed host redhat-srv02.dev.naijalabs.net with IP 192.168.1.204.
Adding host redhat-srv03.dev.naijalabs.net with IP 192.168.1.224...
Next host ID: 10641
Next interface ID: 39
Successfully added IP address 192.168.1.224 for host redhat-srv03.dev.naijalabs.net (host ID: 10641, interface ID: 39).
Successfully processed host redhat-srv03.dev.naijalabs.net with IP 192.168.1.224.
...omitted for brevity...
Now, log on to your Zabbix instance to verify the script executed as expected.
Photo by admingeek from Infotechys
By automating the process of adding hosts to Zabbix with a Bash script, you can save time and reduce errors. This script is a powerful tool for system administrators and engineers managing multiple hosts. With its simple structure, you can easily modify it to suit your specific needs.
Did you find this article useful? Your feedback is invaluable to us! Please feel free to share your thoughts in the comments section below.
In this article, we will explore how to implement security warning banners in Zabbix to enhance compliance and user awareness. This step-by-step guide covers creating
This blog post covers how to install and configure Zabbix version 7.0-2 on RHEL 9 in detail, including prerequisites, step-by-step instructions, and troubleshooting tips. Table
Automating Zabbix Installations Using Ansible simplifies the deployment and configuration of Zabbix, an open-source monitoring solution. This comprehensive guide provides step-by-step instructions, essential YAML configurations,