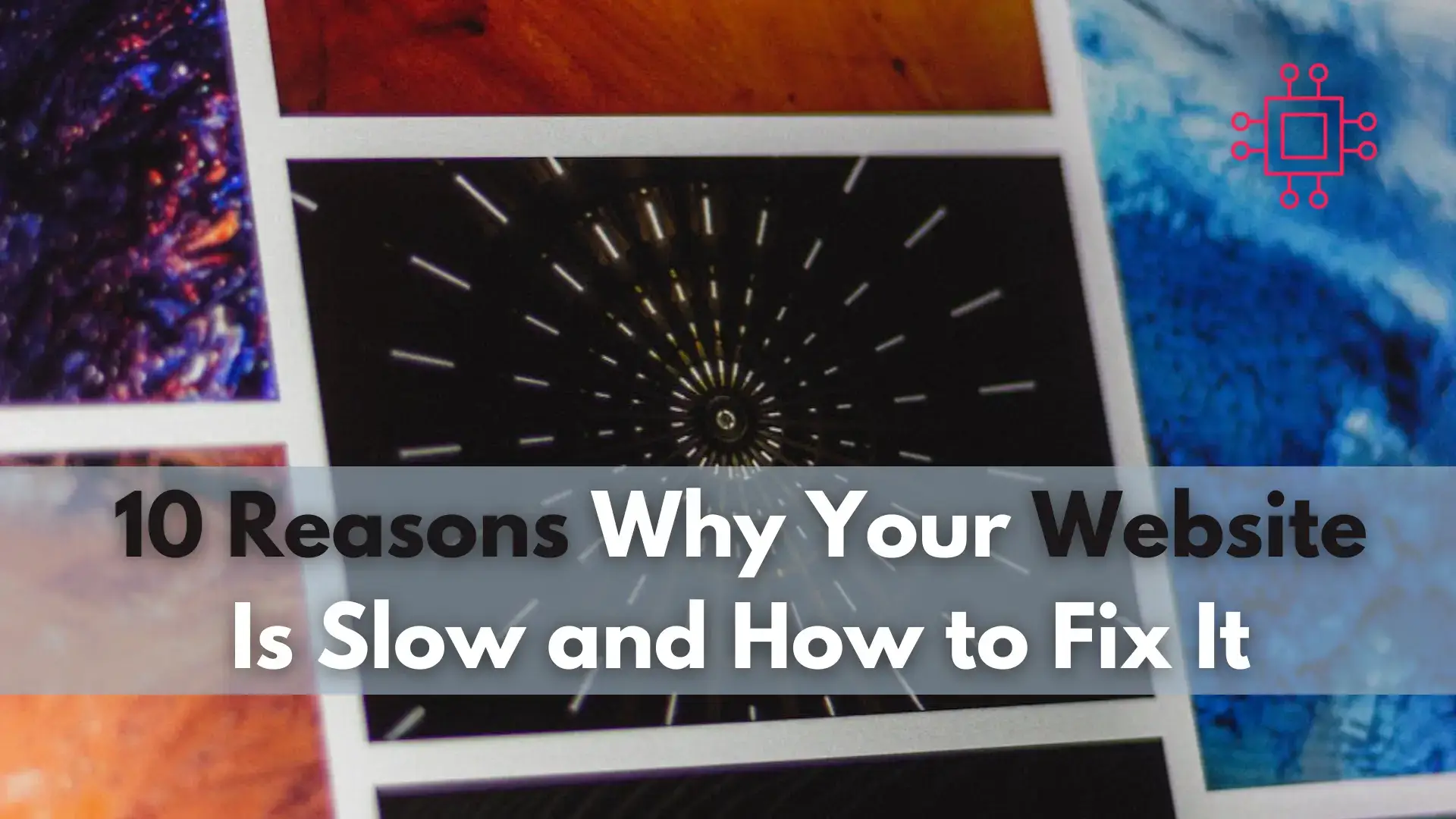
To ensure your website performs optimally, let’s delve into 10 reasons why your website is slow and how to fix it. Table of Contents Introduction
In this tutorial, we’ll explore how to leverage Python to convert PNG images to WebP format, empowering you to enhance your website’s performance and boost its search engine ranking.
In today’s digital age, where online presence is paramount, website optimization stands as a cornerstone for ensuring fast load times and providing users with seamless browsing experiences. As websites become increasingly complex with multimedia elements and rich content, optimizing every aspect becomes crucial for maintaining performance standards. Among these optimization strategies, image compression plays a pivotal role. Images often constitute a significant portion of a web page’s content, and optimizing their file sizes can drastically improve load times and overall page performance.
Image compression is the process of reducing the size of an image file without significantly compromising its visual quality. By minimizing file sizes, websites can deliver content more efficiently, resulting in faster loading times and improved user engagement. While there are various image formats available, each with its own compression algorithms and trade-offs, WebP has emerged as a highly efficient solution.
WebP, developed by Google, is an innovative image format designed specifically for the web. It employs advanced compression techniques to achieve significantly smaller file sizes compared to traditional formats like JPEG and PNG, while preserving image quality. This means that websites can deliver high-quality images at faster speeds, enhancing user experiences across devices and network conditions.
One of the key advantages of WebP is its support for both lossy and lossless compression modes. Lossy compression reduces file sizes by discarding some image data, resulting in a slight reduction in quality that is often imperceptible to the human eye. Lossless compression, on the other hand, retains all image data while still achieving impressive compression ratios, making it ideal for preserving image quality in scenarios where pixel-perfect accuracy is required.
Moreover, WebP supports features like transparency and animation, making it a versatile choice for a wide range of web content. With its ability to handle diverse image types effectively, WebP enables web developers and designers to optimize their websites for performance without compromising on visual appeal.
Before embarking on the journey of optimizing your website’s images, make sure you have the following prerequisites in place:
This tutorial assumes a Linux environment, so make sure you have a fresh installation of Red Hat Enterprise Linux (RHEL), CentOS, or Ubuntu. Additionally, ensure you have root access or sudo privileges to download and install packages on your system.
Python is a fundamental requirement for the image conversion script. Check if Python is installed by running the following commands:
$ sudo dnf install python3
$ sudo apt install python3
This will install the python3 package on your machine.
The Pillow
library is a powerful tool for image processing in Python. Install it using the following command:
$ sudo apt install python3-pip
$ pip3 install pillow
$ sudo apt install python3-pip
$ pip3 install pillow
These commands install the Python package manager (pip3
) and then use it to install the Pillow
library.
Ensure you have PNG image files that you want to convert to WebP format. Place these files in a directory, and make a note of the directory path as you’ll need it later. For demonstration purposes, we’ve created a png-files
directory and populated it with three PNG files each 1.6MB
in size (shown below):
[admin@node1 ~]$ ls -lh png-files/
total 4.8M
-rw-r--r--. 1 admin admin 1.6M Feb 6 00:40 pic1.png
-rw-r--r--. 1 admin admin 1.6M Feb 6 00:40 pic2.png
-rw-r--r--. 1 admin admin 1.6M Feb 6 00:40 pic3.png
[admin@node1 ~]$
Now that you have the prerequisites in place, you’re ready to proceed with the step-by-step process of converting PNG image formats to WebP using Python.
Now, let’s create a Python script to handle the conversion process. We’ll call it convert_to_webp.py
. Open your favorite text editor and paste the following code:
import sys
from PIL import Image
import os
def convert_to_webp(input_path, output_path):
try:
image = Image.open(input_path)
image.save(output_path, 'webp')
print(f"Conversion successful! WebP image saved as {output_path}")
except FileNotFoundError:
print("Error: The specified file does not exist.")
def main():
if len(sys.argv) != 2:
print("Usage: python3 convert_to_webp.py ")
sys.exit(1)
input_path = sys.argv[1]
if input_path.endswith('.png'):
output_path = os.path.splitext(input_path)[0] + '.webp'
convert_to_webp(input_path, output_path)
else:
print("Error: Please provide a PNG file as input.")
if __name__ == "__main__":
main()
In summary, this script takes the path to a PNG file as an argument, converts the PNG file to WebP format, and saves the converted file in the same directory with the same name but a ‘.webp’ extension. It provides error handling for cases where the specified file does not exist or is not a PNG file.
Let’s break down the script and explain each part:
import sys
from PIL import Image
import os
Here, we import necessary modules:
sys
: This module provides access to some variables used or maintained by the Python interpreter and to functions that interact with the interpreter.Image
from PIL
: This is the main module for the Python Imaging Library (PIL), which provides image processing capabilities.os
: This module provides a way of using operating system-dependent functionality.
def convert_to_webp(input_path, output_path):
try:
image = Image.open(input_path)
image.save(output_path, 'webp')
print(f"Conversion successful! WebP image saved as {output_path}")
except FileNotFoundError:
print("Error: The specified file does not exist.")
convert_to_webp
takes two arguments: input_path
(the path to the input PNG file) and output_path
(the path where the converted WebP file will be saved).Image.open()
from the PIL library..save()
method, specifying the output path and ‘webp’ format.FileNotFoundError
exception and prints an error message.
def main():
if len(sys.argv) != 2:
print("Usage: python3 convert_to_webp.py ")
sys.exit(1)
input_path = sys.argv[1]
if input_path.endswith('.png'):
output_path = os.path.splitext(input_path)[0] + '.webp'
convert_to_webp(input_path, output_path)
else:
print("Error: Please provide a PNG file as input.")
main()
function serves as the entry point of the script.sys.argv
).os.path.splitext()
and concatenation.convert_to_webp()
function with the input and output paths.
if __name__ == "__main__":
main()
main()
function is executed only if the script is run directly, not if it is imported as a module into another script.main()
function to start the execution of the script.Once you’ve saved the script, navigate to the directory containing it in your terminal and execute it using Python:
$ python3 convert_to_webp.py
Here an example of the conversion script in action. We’ve executed the script against the PNG files in our png-files
directory (output below).
[admin@node1 ~]$ python3 convert_to_webp.py png-files/pic1.png; python3 convert_to_webp.py png-files/pic2.png; python3 convert_to_webp.py png-files/pic3.png
Conversion successful! WebP image saved as png-files/pic1.webp
Conversion successful! WebP image saved as png-files/pic2.webp
Conversion successful! WebP image saved as png-files/pic3.webp
Notice the dramatic difference in size between the WebP and PNG files.
[admin@node1 ~]$ ls -lh png-files
total 4.9M
-rw-r--r--. 1 admin admin 1.6M Feb 6 00:40 pic1.png
-rw-r--r--. 1 admin admin 55K Feb 6 02:05 pic1.webp
-rw-r--r--. 1 admin admin 1.6M Feb 6 00:40 pic2.png
-rw-r--r--. 1 admin admin 47K Feb 6 02:05 pic2.webp
-rw-r--r--. 1 admin admin 1.6M Feb 6 00:40 pic3.png
-rw-r--r--. 1 admin admin 46K Feb 6 02:05 pic3.webp
Converting PNG image formats to WebP using Python is a straightforward process that can significantly enhance your website’s performance. By following the steps outlined in this tutorial, you’ll be equipped to optimize your images efficiently, resulting in faster load times and improved user experiences.
Implementing image optimization techniques like WebP conversion demonstrates your commitment to providing a seamless browsing experience for your website visitors. As search engines prioritize fast-loading websites, optimizing your images with WebP can also positively impact your search engine ranking.
Did you find this article useful? Your feedback is invaluable to us! Please feel free to share your thoughts in the comments section below.
Related Posts
To ensure your website performs optimally, let’s delve into 10 reasons why your website is slow and how to fix it. Table of Contents Introduction
Have you ever wondered how search engines gather all that information from the internet? Learn how to build your own web crawler in Python and