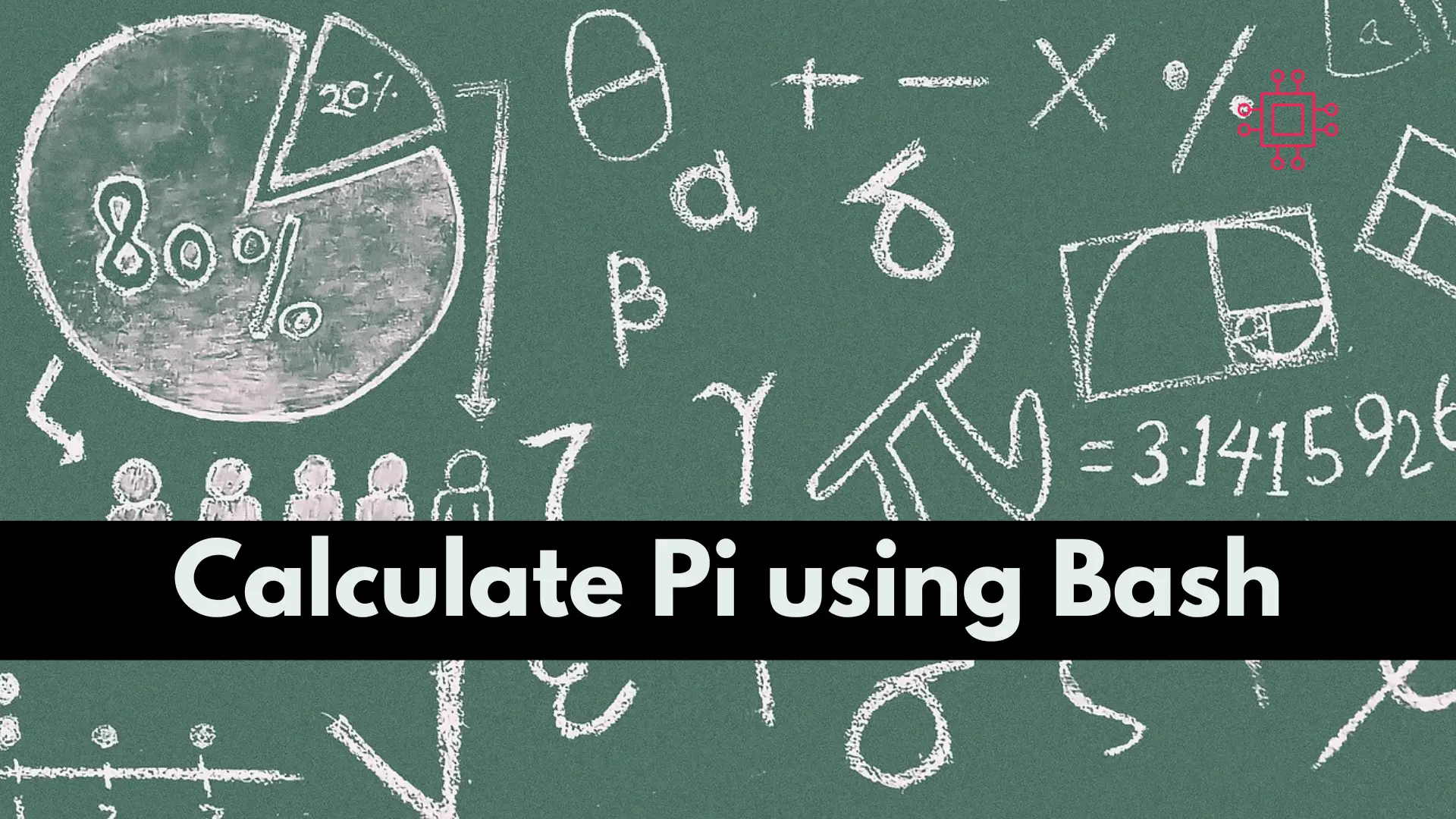
To calculate pi using a Bash script, you can use the bc command, which is a tool that can perform arithmetic operations in the terminal.
Learn how to generate secure, random passwords with a Bash script. This step-by-step guide will help you create customizable passwords for better security.
In today’s digital world, password security is more important than ever. From personal accounts to corporate logins, passwords are our first line of defense against cyber threats. Whether you’re a developer, system administrator, or just someone looking to secure your online presence, generating strong, random passwords is essential.
In this blog post, we will explore how to generate secure, random passwords using a simple Bash script. We will also dive into best practices, considerations for password security, and how you can customize the script to fit your needs. If you’re looking to enhance your password management strategy, this guide will help you get started quickly and efficiently.
Why Password Security Matters |
Before we dive into the script, let’s quickly review why password security is paramount. Weak passwords are one of the most common entry points for cybercriminals. Hackers use a variety of techniques such as brute force attacks and dictionary attacks to crack passwords. Therefore, using strong, random passwords that are difficult to guess or crack is crucial for safeguarding sensitive information.
Here are some key principles of a secure password:
Length: The longer the password, the more difficult it is to crack.
Complexity: A mix of uppercase and lowercase letters, numbers, and special characters makes a password harder to guess.
Uniqueness: Each password should be unique, avoiding reuse across different accounts.
This is where the Bash script comes in—automating the generation of strong, random passwords based on your specific criteria.
The Bash script we’ll cover in this blog allows you to generate secure passwords with a minimum of 8 characters. It also gives you flexibility in specifying the number of passwords to generate and their desired length.
Let’s go over the steps involved in creating this script, how it works, and what you can do to modify it according to your needs.
Understanding the Script |
Here is the complete Bash script that generates random passwords:
#!/bin/bash
# Function to generate a random password
generate_password() {
local length=$1
# Define the character set for the password
local char_set="A-Za-z0-9!@#$%^&*()-_=+[]{}|;:,.<>?"
# Generate a random password using the specified length
tr -dc "$char_set" < /dev/urandom | head -c "$length"
echo
}
# Check if the number of arguments is correct
if [ "$#" -ne 2 ]; then
echo "Usage: $0 "
exit 1
fi
# Assign command line arguments to variables
password_length=$1
num_passwords=$2
# Ensure the password length is at least 8 characters
if [ "$password_length" -lt 8 ]; then
echo "Password length must be at least 8 characters."
exit 1
fi
# Generate the specified number of passwords
for ((i=1; i<=$num_passwords; i++)); do
generate_password "$password_length"
done
Breaking Down the Script |
Let’s break down the different parts of the script to understand how it works:
Function to Generate Passwords |
generate_password
is the function that takes alength
argument and generates a random password using characters from the character setA-Za-z0-9!@#$%^&*()-_=+[]{}|;:,.<>?
.
It uses
/dev/urandom
, a special file that provides random data, and thetr
command to filter the allowed characters. Thehead
command ensures that only the specified number of characters are selected.
Argument Checking |
The script expects two arguments: the password length and the number of passwords to generate. If either of these is missing or invalid, the script will display a usage message and exit.
Password Length Validation |
The script ensures that the password length is at least 8 characters. If the user provides a length shorter than this, an error message is shown.
Loop for Multiple Passwords |
If the user specifies that they want multiple passwords, the script will loop the desired number of times and generate the specified number of passwords.
Photo by admingeek from Infotechys
A strong password is one that is difficult for attackers to guess or brute force. Here’s a breakdown of factors that contribute to strong passwords:
Factor | Description |
---|---|
Length | Passwords should be at least 12 characters long. Longer passwords are harder to crack. |
Character Variety | Use a mix of uppercase and lowercase letters, numbers, and special characters to increase complexity. |
Unpredictability | Avoid using predictable patterns or common words. Use random combinations of characters instead. |
Uniqueness | Don’t reuse passwords across multiple sites. Each account should have its own unique password. |
By using the Bash script outlined earlier, you can ensure that your passwords meet these security criteria.
Generating Secure Passwords Using the Script |
Once you’ve created the script and saved it as a .sh
file, you can run it from your terminal with the following syntax:
./generate_password.sh
Example 1: Generating 5 passwords of 12 characters |
./generate_password.sh 12 5
tYOHVqe:r3AX
u*WaQ+3yH$9m
w7j@BZ2oM1Kl
D9P&kVz8Lb36
T^7vR*Lmn1Fx
Example 2: Generating 3 passwords of 16 characters |
./generate_password.sh 16 3
g#jXk9!y7^9QpD+z
T2q$u^dRZFmP*4Lh
s$wM
As you can see from the examples above, each password is random and contains a mix of uppercase and lowercase letters, numbers, and special characters.
While many password managers can generate random passwords, using a custom Bash script offers a few unique advantages:
Customization: You can adjust the script to fit your exact needs (e.g., changing the character set or adding additional security features).
Automation: Once set up, the script can be run automatically as part of your system maintenance routine.
No Dependencies: The script uses tools (
tr
,head
,/dev/urandom
) that are native to most Unix-like systems, so you don’t need to install any additional software.
Enhancements You Can Make to the Script |
While the script as it is provides a good starting point, you can add more functionality based on your needs. Here are a few enhancements you could consider:
Include Password Expiry: Implement a feature that generates passwords that expire after a certain period.
Hashing: Add the option to hash the generated passwords before saving them, enhancing security further.
Output to File: Modify the script to save the generated passwords to a file, useful for bulk password creation.
Strength Check: Integrate a password strength checker that validates if the generated password is strong enough.
Generating strong, random passwords is only the first step. It’s important to follow best practices for managing and using those passwords:
Use a Password Manager: Store your passwords in a secure password manager to avoid the temptation of writing them down or reusing them.
Enable Multi-Factor Authentication (MFA): Whenever possible, enable MFA for added security.
Regularly Update Passwords: Periodically change your passwords and ensure they remain unique and strong.
By using the Bash script we discussed in this post, you can generate secure, random passwords with ease. This script provides you with the flexibility to customize your password generation needs while ensuring that your passwords meet the criteria for strength and security.
Remember, the best security practices go beyond generating strong passwords—they include proper management and regular updates. If you’re looking to bolster your cybersecurity efforts, automating your password generation is an excellent place to start.
Did you find this article useful? Your feedback is invaluable to us! Please feel free to share this post! And, your thoughts in the comments section below.
To calculate pi using a Bash script, you can use the bc command, which is a tool that can perform arithmetic operations in the terminal.
Learn 10 best practices for Bash scripting and start writing scripts that are efficient and maintainable. This guide covers meaningful variable names, error handling, quoting
Learn how to automate adding hosts to Zabbix using Bash script. This comprehensive guide covers script functions, examples, and best practices to streamline your IT