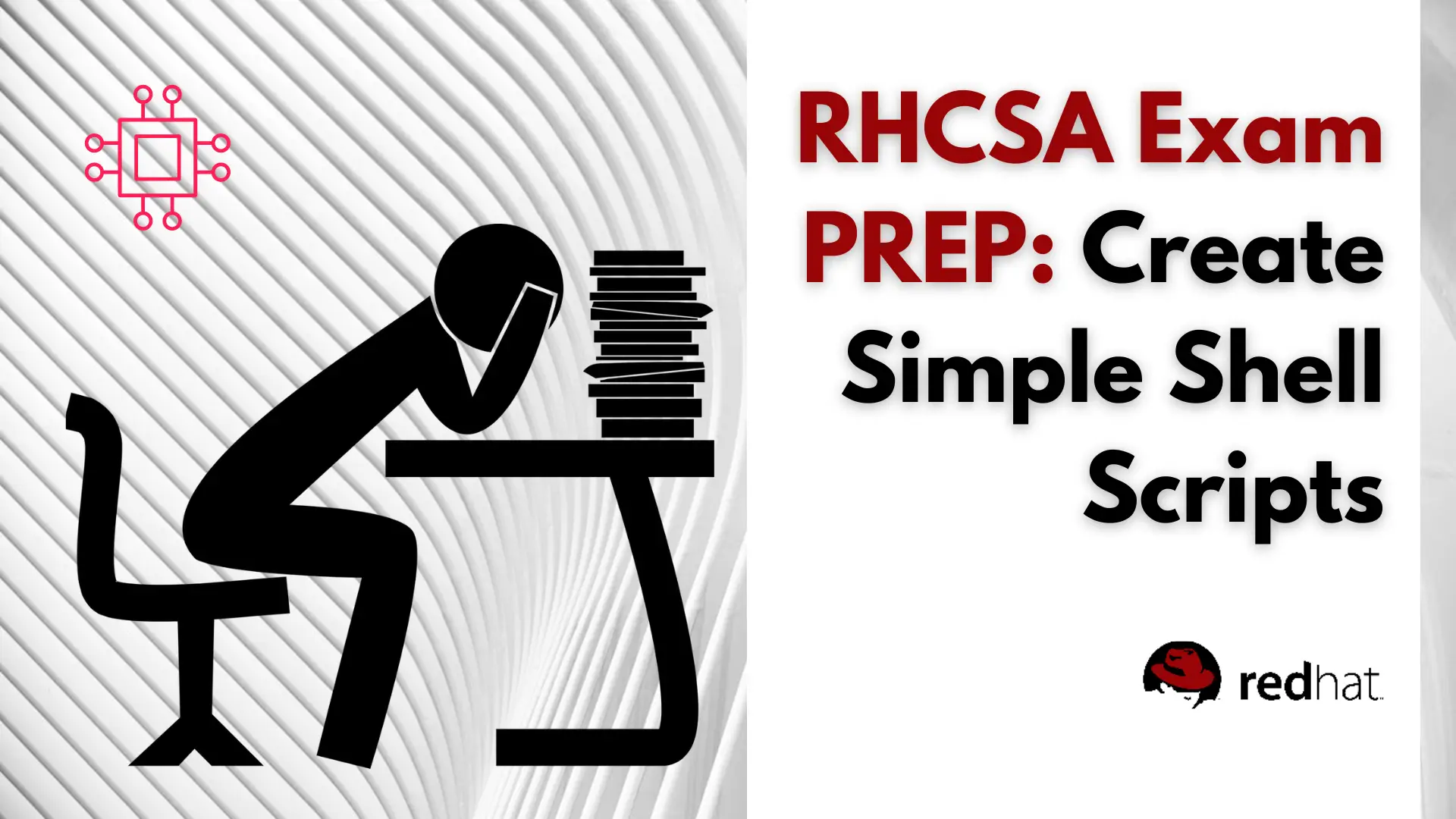
Are you preparing for the RHCSA 9 exam and want to master the essential skill of shell scripting? Discover 10 practical shell scripts that can
Learn 10 best practices for Bash scripting and start writing scripts that are efficient and maintainable. This guide covers meaningful variable names, error handling, quoting variables, logging, and more to help you improve your Bash scripting skills.
Bash scripting is a powerful tool for automating tasks in Linux environments, making it indispensable for systems administrators, DevOps engineers, and developers alike. However, crafting effective and maintainable bash scripts requires adherence to best practices. In this post, we’ll explore 10 essential best practices for bash scripting that will help you create scripts that are robust, efficient, and scalable.
Meaningful variable names improve the readability and maintainability of your scripts. Avoid single-letter variables unless used in simple loops or where their purpose is clear.
# Bad practice:
x=10
# Good practice:
num_files=10
Using descriptive names helps others (and future you) understand what the script is doing.
Adding comments to your scripts improves readability and provides context. Clear documentation helps others understand the purpose of your script and what each section does.
#!/bin/bash
# This script cleans up log files older than 7 days in the /var/log directory
find /var/log -type f -name "*.log" -mtime +7 -exec rm {} \;
Tip: Use a standardized header comment block to describe the script’s purpose, author, and usage.
#!/bin/bash
#
# Script Name: log_cleanup.sh
# Description: This script removes log files older than 7 days from the /var/log directory.
# Author: Jane Doe
# Date: 2024-08-15
# Version: 1.0
#
# Usage:
# ./log_cleanup.sh
#
# Parameters:
# None
#
# Exit Status:
# 0 - Success
# 1 - Failure
#
# Example:
# To execute the script, simply run:
# ./log_cleanup.sh
#
# Notes:
# - Make sure you have the correct permissions to delete files in the /var/log directory.
# - Adjust the retention period by modifying the '-mtime +7' option.
This header block (above) provides an overview of the script, making it easier for others to understand its purpose and usage.
Enabling strict mode helps to catch common errors early by terminating the script on errors and undefined variables.
#!/bin/bash
set -euo pipefail
Option | Description |
---|---|
set -e | Stops script execution on errors. |
set -u | Treats unset variables as errors. |
set -o pipefail | Prevents errors in a pipeline from being masked. |
Always use quotes around your variables to avoid word splitting and globbing issues, especially when dealing with file names that may contain spaces.
This simple habit prevents errors that could disrupt your script’s functionality.
# Bad practice:
rm $file_name
# Good practice:
rm "$file_name"
Functions allow you to encapsulate code blocks, making scripts modular and easier to debug or update.
#!/bin/bash
function backup_logs {
tar -czf backup_$(date +%Y-%m-%d).tar.gz /var/log/*.log
}
backup_logs
This makes your code more modular and reusable across different scripts.
Always check the exit status of commands, especially when writing scripts that manage critical processes. Exit codes help determine if a command succeeded or failed, allowing you to handle errors more gracefully.
if cp /important/file /backup/; then
echo "Backup successful"
else
echo "Backup failed" >&2
exit 1
fi
Exit codes ensure that failures don’t go unnoticed.
Instead of using space-separated strings for lists, use arrays. Arrays are more flexible and allow for better manipulation of data.
files=("file1.txt" "file2.txt" "file3.txt")
for file in "${files[@]}"; do
echo "Processing $file"
done
This approach ensures safer handling of complex data structures.
Global variables can cause unexpected behaviors, especially in large scripts. Use local variables within functions to limit their scope and reduce potential side effects.
#!/bin/bash
function greet {
local name="John"
echo "Hello, $name"
}
Limiting the scope of variables minimizes conflicts and unintended overwrites.
ShellCheck is an essential tool for static analysis of bash scripts. It can detect bugs and provide suggestions for improvement.
#!/bin/bash
echo "Enter your name:"
read name
echo "Hello, $name"
Before running your scripts in production, run them through ShellCheck to catch errors early.
Logging is essential for debugging and monitoring. Include logging mechanisms to track script behavior over time, especially in production environments.
#!/bin/bash
log_file="/var/log/myscript.log"
echo "$(date) - Script started" >> "$log_file"
This ensures that you have a record of your script’s execution, which is crucial when troubleshooting issues.
By following these 10 best practices, you’ll be able to write bash scripts that are reliable, maintainable, and easy to troubleshoot. Adopting these habits will not only improve your scripting but also enhance the robustness of your production systems. Moreover, these techniques will give your scripts the polish and efficiency needed for optimal performance.
Did you find this article useful? Your feedback is invaluable to us! Please feel free to share your thoughts in the comments section below.
Related Posts
Are you preparing for the RHCSA 9 exam and want to master the essential skill of shell scripting? Discover 10 practical shell scripts that can
In this tutorial, we’ll explore how to leverage Python to convert PNG images to WebP format, empowering you to enhance your website’s performance and boost
To calculate pi using a Bash script, you can use the bc command, which is a tool that can perform arithmetic operations in the terminal.