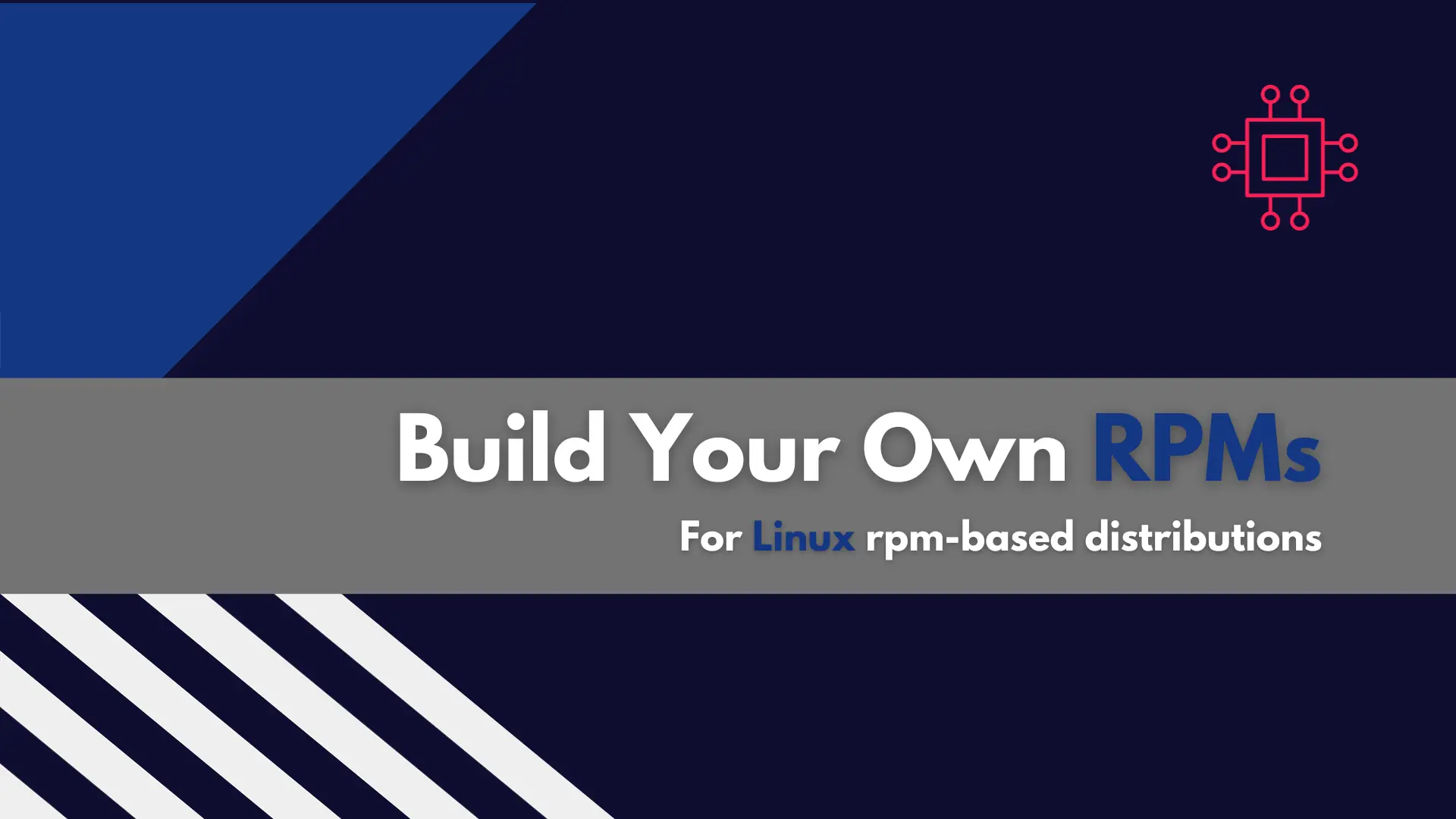
With our step-by-step guide, you can easily build your own RPM packages in Linux on RHEL and CentOS, and efficiently manage software updates and installations.
Do you want to build your own mortgage calculator with just a few lines of code? Learn how to use shortcodes to create a custom mortgage calculator on your website!
If you are a website owner looking to add a mortgage calculator to your WordPress site, you’re in luck. With the power of shortcodes, you can easily create a mortgage calculator that allows users to estimate their monthly payments. In this article, we’ll break down the HTML code for a simple mortgage calculator and show you how to turn it into a shortcode that you can add to your site (shown below).
A shortcode is a small piece of code that allows you to add dynamic functionality to your WordPress site. Shortcodes are enclosed in square brackets, like this: [shortcode]
. When WordPress encounters a shortcode on a page or post, it replaces the shortcode with a dynamic piece of content.
Shortcodes are incredibly versatile and can be used to add a wide range of functionality to your site, including contact forms, photo galleries, and even e-commerce functionality. One of the most common uses for shortcodes is to create custom forms, like a mortgage calculator.
Shortcodes were introduced in WordPress version 2.5 in 2008. Since then, they have become an essential tool for developers and website owners alike. Shortcodes make it easy to add complex functionality to a website without requiring extensive coding knowledge. Today, shortcodes are an integral part of the WordPress ecosystem and are used by millions of websites worldwide.
Let’s take a closer look at the HTML code for a simple mortgage calculator. This code provides a basic framework for a mortgage calculator that allows users to input their home value, down payment, interest rate, and loan term. It also includes a button to calculate the monthly payment and a read-only field to display the result.
If you copy and paste the following code into your shortcode content box, your webpage will display something similar to the mortgage calculator on this page. The color scheme will adapt with the theme you’re using for your website.
So, without further ado, here’s a copy of the html code:
Mortgage Calculator
Mortgage Calculator
The <head>
section contains the document’s metadata, such as its title, author, and links to CSS stylesheets and JavaScript files.
Mortgage Calculator
In this code snippet, we only have the title tag. The title tag defines the title of the document, which is displayed in the browser’s title bar or tab.
The <body>
section contains the main content of the web page. In this section, we have the following:
Mortgage Calculator
This is a simple heading that displays the text “Mortgage Calculator” in a larger font size.
This is the main section of the web page where users can input data and perform calculations. The form contains the following input fields:
home-value
: The user inputs the home value in this field. The required
attribute ensures that the user must input a value before submitting the form.down-payment
: The user inputs the down payment in this field. The required
attribute ensures that the user must input a value before submitting the form.interest-rate
: The user inputs the interest rate in this field. The required
attribute ensures that the user must input a value before submitting the form.loan-term
: The user selects the loan term from a dropdown list in this field. The required
attribute ensures that the user must select an option before submitting the form.monthly-payment
: This field displays the calculated monthly payment. The readonly
attribute ensures that the user cannot modify the value manually.
This is the button that the user clicks to calculate the monthly payment. The onclick
attribute calls the calculate()
function in the JavaScript section.
This is the main function that is responsible for calculating the monthly payment based on the user’s input. The function is triggered by clicking the “Calculate” button, which has an onclick
attribute that calls the calculate()
function.
The calculate()
function first gets the user input values for home value, down payment, interest rate, and loan term using document.getElementById()
. It then calculates the monthly payment using the formula for mortgage payments, which is (P * r * (1 + r)^n) / ((1 + r)^n - 1)
, where P
is the principal amount (home value minus down payment), r
is the monthly interest rate, and n
is the number of monthly payments (loan term times 12).
The monthly payment is then assigned to the value
attribute of the monthly-payment
input element using document.getElementById()
. The value is formatted to display a dollar sign and two decimal places using toFixed()
.
That’s it! We’ve thoroughly reviewed how to build a custom mortgage calculator using shortcodes. You can incorporate this HTML code into your shortcode content to create a simple mortgage calculator that looks similar to the one shown on this page. Or, customize the code to develop a more interactive calculator with additional features.
When creating a mortgage calculator or any other form that takes user input, it’s essential to follow some best practices:
Shortcodes are a powerful tool in WordPress that make it easy to add dynamic functionality to your website. By using shortcodes, you can quickly and easily create a mortgage calculator that allows users to estimate their monthly payments. When building your calculator, be sure to follow best practices to ensure that your form is easy to use, accessible, and visually appealing.
Related Posts
With our step-by-step guide, you can easily build your own RPM packages in Linux on RHEL and CentOS, and efficiently manage software updates and installations.
Have you ever wondered how search engines gather all that information from the internet? Learn how to build your own web crawler in Python and
In this tutorial, you will learn the process involved with building a docker registry. As part of this process, we will set up a web